by Ange Tuteur July 14th 2017, 4:16 pm
@Wolfuryo when using match, you need to check that it isn't null before accessing the indexes of the returned array otherwise it throws an error. ex :
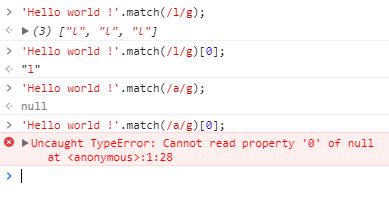
So for example, instead of this :
- Code:
var d = $(".vote", data).eq(0).html();
var ls = /vote-no-bar/.test(d) ? 0 : d.match(/\d+ votes|\d+ vote/)[0].match(/\d+/)[0];
$("a.topictitle").eq(l).after(likes.config.html.replace(/%likes/g, ls));
l++;
likes.gett(l);
You can do this :
- Code:
var d = $(".vote", data).eq(0).html(),
ls,
match;
if (/vote-no-bar/.test(d)) {
ls = 0
} else {
match = d.match(/\d+ votes|\d+ vote/);
if (match && match[0]) {
match = match[0].match(/\d+/);
if (match && match[0]) {
ls = match[0]
}
}
}
$("a.topictitle").eq(l).after(likes.config.html.replace(/%likes/g, ls));
l++;
likes.gett(l);
It's a little more statements, but it should ensure that you don't get any errors when making matches. You can also use test() to search what you're looking for as well, because it only returns a boolean value and it should cut down on the size a bit. Match is good for certain instances (other times it's slower), but you have to be careful when using it, because you can easily throw errors.
Personally, I like to use replace() along with capture groups to get the values I'm looking for. It's pretty much the same thing, only the prior method doesn't require you to access indexes which could be null. (see below)
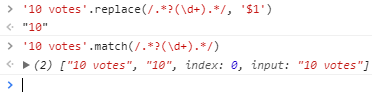